728x90
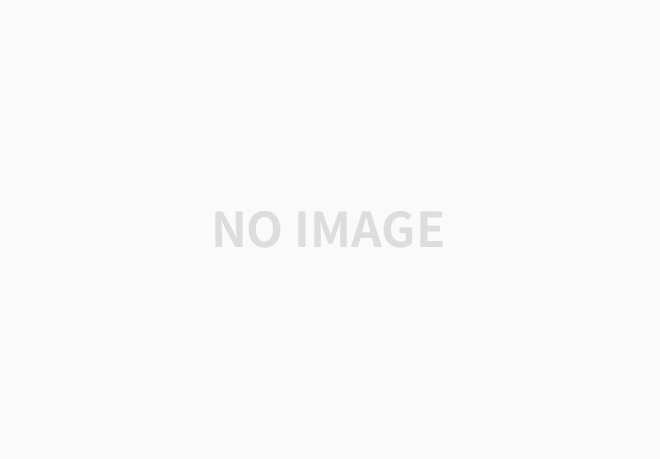
<script>
const 함수 = function() {
alert('함수_01');
alert('함수_02');
}
const sum = 0;
console.log(함수);
함수(); //함수 실행
console.log(typeof(함수)); //함수 타입이 뭔지.
console.log(typeof(sum));
function 함수02(){
alert('함수02_01');
alert('함수02_02');
}
console.log(함수02);
함수02();
console.log(typeof(함수02));
</script>
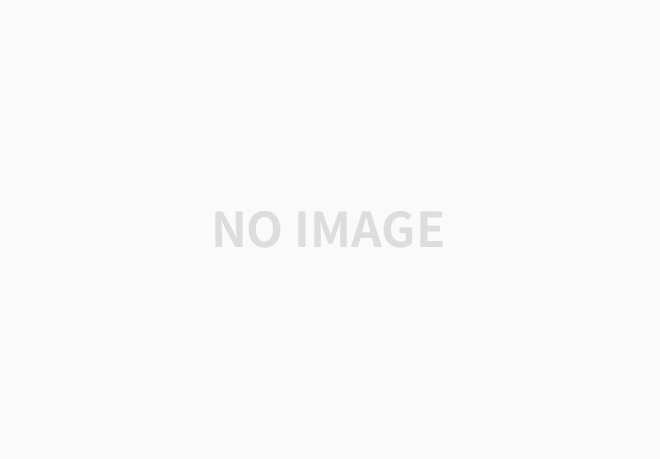
<script>
//함수선언
function f(x){
return x * x;
}
//함수 호출
f(30);
console.log(f(30));
</script>
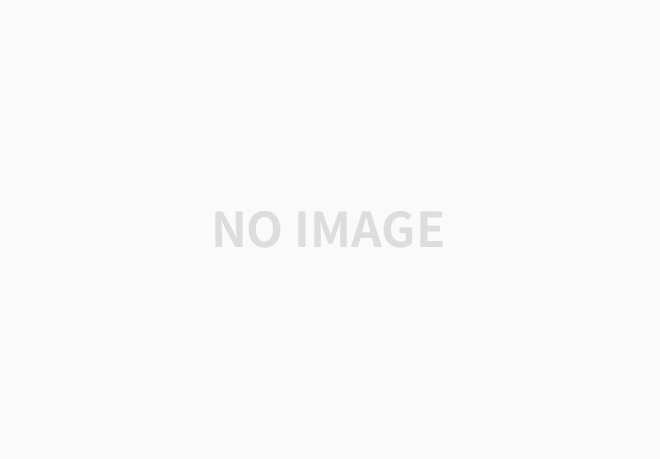
callback함수
<script>
var callback = function () {
console.log('callback함수임');
}
function callTenTimes(abc) {
for (let i = 0; i < 10; i++) {
console.log(i);
abc();
}
}
callTenTimes(callback);
callback();
callTenTimes(function(){
console.log('바로 호출');
});
</script>
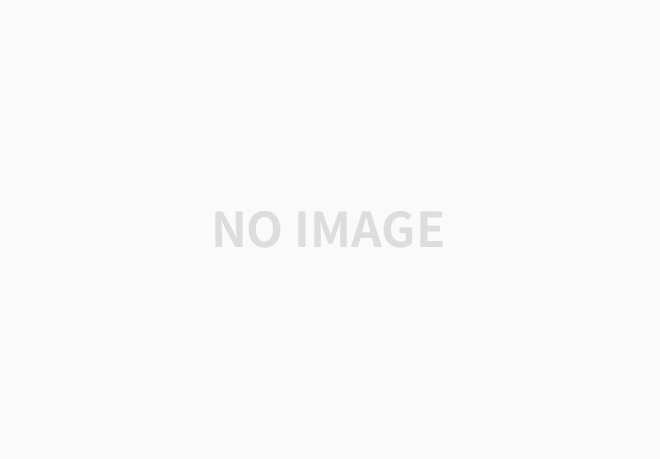
객체
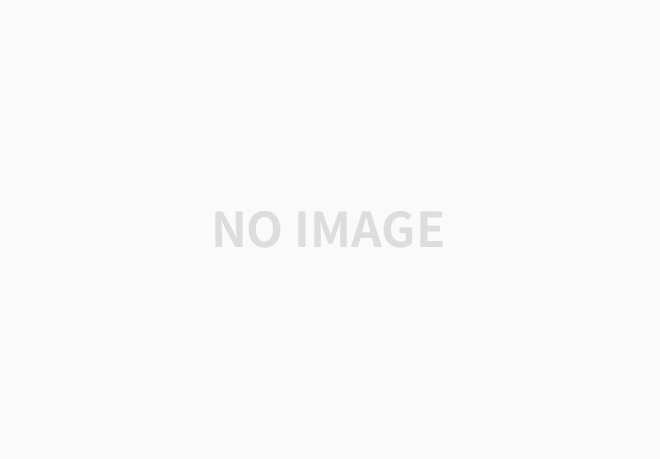
이렇게 key 값을 줘야한다.
<script>
var product = {
제품명 : '건조 망고',
유형 : '당절임',
성분 : '망고, 설탕, 메타중아황산나트륨, 치자황색소',
원산지 : '필리핀'
};
for (var i in product){
//alert(i + ':' + product[i]);
console.log(i + ':' + product[i]);
};
</script>
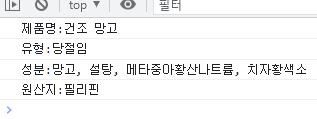
<script>
const person ={
name : '홍길동',
eat : function(food){
console.log(this.name + '이 ' + food + '를 먹습니다.');
}
}
person.eat('피자');
person.eat('햄버거');
console.log(person.name);
</script>
</script>
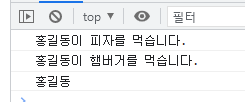
<script>
document.querySelector('h1').style.backgroundColor = 'red';
document.querySelector('h2').style.color = 'red';
</script>
<body>
<h1>Process - 1</h1>
<h2>Process - 2</h2>
</body>

순서를 바꿔준다.
<body>
<h1>Process - 1</h1>
<h2>Process - 2</h2>
</body>
<script>
document.querySelector('h1').style.backgroundColor = 'red';
document.querySelector('h2').style.color = 'red';
</script>
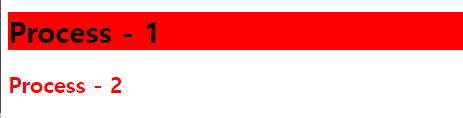
또는
<script>
window.onload = function(){
document.querySelector('h1').style.backgroundColor = 'red';
document.querySelector('h2').style.color = 'red';
}
</script>
<body>
<h1>Process - 1</h1>
<h2>Process - 2</h2>
</body>
문서객체
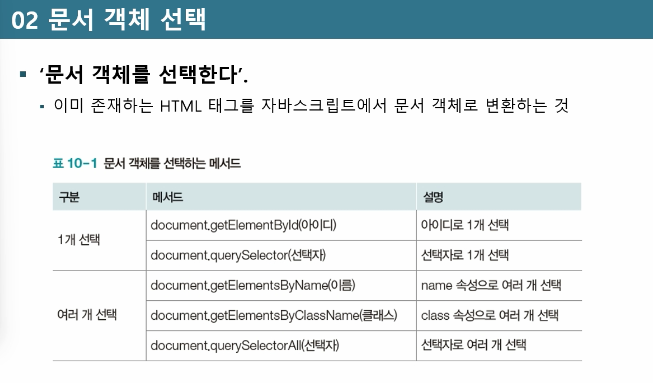
<script>
window.onload = function(){
document.querySelector('h1').style.backgroundColor = 'red';
document.querySelector('h2').style.color = 'red';
const header = document.getElementById('header');
header.style.color = 'orange';
header.style.background = 'blue';
header.innerHTML = 'From Javascript';
const h2 = document.querySelector('h2');
h2.style.color = 'green';
h2.innerHTML = 'From Javascript2';
}
</script>
<body>
<h1>Process - 1</h1>
<h2>Process - 2</h2>
<h1 id="header">Header</h1>
</body>
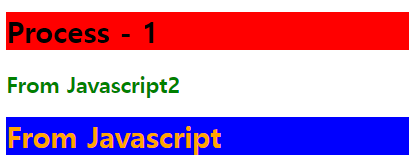
<script>
window.onload = function () {
let output = '';
for (let i = 0; i < 10; i++) {
output += '<h1>header - ' + '<h1>';
}
document.body.textContent = output; // <h1>부터 다 출력
document.body.innerHTML = output; //header - 이부분만 출력
}
</script>
<script>
window.onload = function(){
let output = '';
for(let i = 0; i<256; i++){
output += '<div></div>';
}
document.body.innerHTML = output;
const divs = document.querySelectorAll('div');
for(let i = 0; i<divs.length; i++){
const div = divs[i];
div.style.height ='2px';
div.style.background = 'rgb('+i+','+i+','+i+')';
}
};
</script>
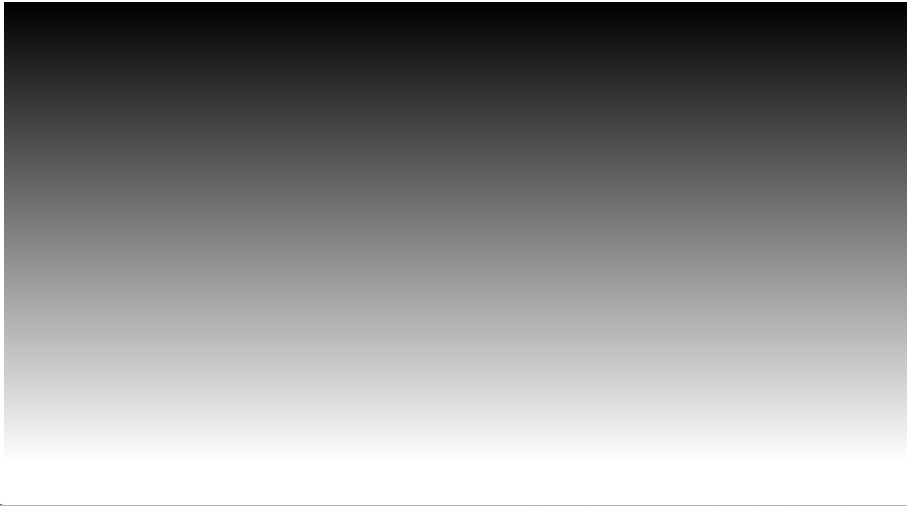
<script>
window.onload = function(){
let image = document.getElementById('image');
image.width = 300;
image.height = 200;
}
</script>
<body>
<img id="image" >
</body>
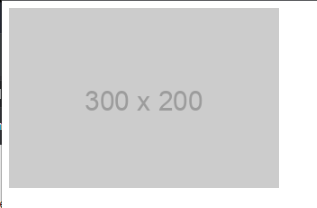
<script>
window.onload = function () {
//속성을 지정
document.body.setAttribute('data-custom', 'value');
//속성을 추출
const data = document.body.getAttribute('data-custom');
console.log(data);
//위에걸 활용해서 시간 추출.
const clock = document.getElementById('clock');
setInterval(function () {
const now = new Date();
clock.innerHTML = now.toString();
}, 1000)
}
</script>
<body>
<h1 id="clock"></h1>
</body>

이벤트
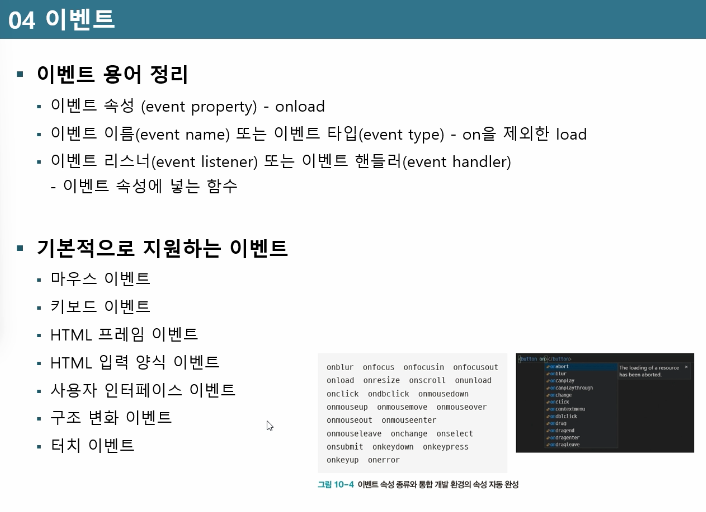
728x90
'웹개발 > HTML' 카테고리의 다른 글
[JavaScript] 제이쿼리 23.04.14 (0) | 2023.04.14 |
---|---|
[JavaScript] 조건문, 반복문 23.04.12 (0) | 2023.04.12 |
[JavaScript] 기본용어 예약어 자료형 변수 23.04.11 (0) | 2023.04.11 |
[HTML] 23.04.10 (0) | 2023.04.10 |
[HTML] 선택자,이미지배경, 박스속성, 가시속성 23.04.06 (0) | 2023.04.06 |
댓글